Note
Go to the end to download the full example code
Benchmarking preprocessing with parallelization and serialization#
In this example, we compare the execution time and memory requirements of
preprocessing data with the parallelization and serialization functionalities
available in braindecode.preprocessing.preprocess()
.
We compare 4 cases:
Sequential, no serialization
Sequential, with serialization
Parallel, no serialization
Parallel, with serialization
Case 1 is the simplest approach, in which all recordings in a
braindecode.datasets.BaseConcatDataset
are preprocessed one after the
other. In this scenario, braindecode.preprocessing.preprocess()
acts
inplace, which means memory usage will likely stay stable (depending on the
preprocessing operations) if recordings have been preloaded. However, two
potential issues arise when working with large datasets: (1) if recordings have
not been preloaded before preprocessing, preprocess() will need to load them
and keep them in memory, in which case memory can become a bottleneck, and (2)
sequential preprocessing can take a considerable amount of time to run when
working with many recordings.
A solution to the first issue (memory usage) is to save the preprocessed data
to a file so it can be cleared from memory before moving on to the next
recording (case 2). The recordings can then be reloaded with preload=False
once they have all been saved to disk. This enables using the lazy loading
capabilities of braindecode.datasets.BaseConcatDataset
and avoids
potential memory bottlenecks. The downside is that the writing to disk can take
some time and of course requires disk space.
A solution to the second issue (slow preprocessing) is to parallelize the preprocessing over multiple cores whenever possible (case 3). This can speed up preprocessing significantly. However, this approach will increase memory usage because of the way parallelization is implemented internally (with joblib, copies of (part of) the data must be made when sending arguments to parallel processes).
Finally, case 4 (combining parallelization and serialization) is likely to be both fast and memory efficient. As shown in this example, this remains a tradeoff though, and the selected configuration should depend on the size of the dataset and the specific operations applied to the recordings.
# Authors: Hubert Banville <hubert.jbanville@gmail.com>
#
# License: BSD (3-clause)
import time
import tempfile
from itertools import product
import pandas as pd
import matplotlib.pyplot as plt
from sklearn.preprocessing import scale
from memory_profiler import memory_usage
from braindecode.datasets import SleepPhysionet
from braindecode.preprocessing import (
preprocess, Preprocessor, create_fixed_length_windows)
We create a function that goes through the usual three steps of data
preparation: (1) data loading, (2) continuous data preprocessing,
(3) windowing and (4) windowed data preprocessing. We use the
braindecode.datasets.SleepPhysionet
dataset for testing purposes.
def prepare_data(n_recs, save, preload, n_jobs):
if save:
tmp_dir = tempfile.TemporaryDirectory()
save_dir = tmp_dir.name
else:
save_dir = None
# (1) Load the data
concat_ds = SleepPhysionet(
subject_ids=range(n_recs), recording_ids=[1], crop_wake_mins=30,
preload=preload)
sfreq = concat_ds.datasets[0].raw.info['sfreq']
# (2) Preprocess the continuous data
preprocessors = [
Preprocessor('crop', tmin=10),
Preprocessor('filter', l_freq=None, h_freq=30)
]
preprocess(concat_ds, preprocessors, save_dir=save_dir, overwrite=True,
n_jobs=n_jobs)
# (3) Window the data
windows_ds = create_fixed_length_windows(
concat_ds, 0, None, int(30 * sfreq), int(30 * sfreq), True,
preload=preload, n_jobs=n_jobs)
# Preprocess the windowed data
preprocessors = [Preprocessor(scale, channel_wise=True)]
preprocess(windows_ds, preprocessors, save_dir=save_dir, overwrite=True,
n_jobs=n_jobs)
Next, we can run our function and measure its run time and peak memory usage for each one of our 4 cases above. We call the function multiple times with each configuration to get better estimates.
Note
To better characterize the run time vs. memory usage tradeoff for your specific configuration (as this will differ based on available hardware, data size and preprocessing operations), we recommend adapting this example to your use case and running it on your machine.
n_repets = 2 # Number of repetitions
all_n_recs = 2 # Number of recordings to load and preprocess
all_n_jobs = [1, 2] # Number of parallel processes
results = list()
for _, n_recs, save, n_jobs in product(
range(n_repets), [all_n_recs], [True, False], all_n_jobs):
start = time.time()
mem = max(memory_usage(
proc=(prepare_data, [n_recs, save, False, n_jobs], {})))
time_taken = time.time() - start
results.append({
'n_recs': n_recs,
'max_mem': mem,
'save': save,
'n_jobs': n_jobs,
'time': time_taken
})
0%| | 0.00/51.1M [00:00<?, ?B/s]
0%| | 65.5k/51.1M [00:00<01:20, 631kB/s]
0%|▏ | 164k/51.1M [00:00<01:04, 786kB/s]
1%|▏ | 295k/51.1M [00:00<00:51, 979kB/s]
1%|▎ | 426k/51.1M [00:00<00:47, 1.06MB/s]
1%|▍ | 557k/51.1M [00:00<00:45, 1.11MB/s]
1%|▍ | 668k/51.1M [00:00<00:49, 1.02MB/s]
2%|▌ | 819k/51.1M [00:00<00:43, 1.16MB/s]
2%|▋ | 950k/51.1M [00:00<00:43, 1.17MB/s]
2%|▊ | 1.08M/51.1M [00:00<00:42, 1.17MB/s]
2%|▊ | 1.20M/51.1M [00:01<00:42, 1.17MB/s]
3%|▉ | 1.33M/51.1M [00:01<00:43, 1.15MB/s]
3%|█ | 1.46M/51.1M [00:01<00:42, 1.17MB/s]
3%|█▏ | 1.58M/51.1M [00:01<00:42, 1.15MB/s]
3%|█▏ | 1.70M/51.1M [00:01<00:42, 1.15MB/s]
4%|█▎ | 1.84M/51.1M [00:01<00:42, 1.16MB/s]
4%|█▍ | 1.97M/51.1M [00:01<00:41, 1.18MB/s]
4%|█▌ | 2.10M/51.1M [00:01<00:41, 1.19MB/s]
4%|█▌ | 2.23M/51.1M [00:01<00:41, 1.19MB/s]
5%|█▋ | 2.36M/51.1M [00:02<00:41, 1.18MB/s]
5%|█▊ | 2.49M/51.1M [00:02<00:41, 1.18MB/s]
5%|█▉ | 2.62M/51.1M [00:02<00:41, 1.17MB/s]
5%|█▉ | 2.75M/51.1M [00:02<00:41, 1.17MB/s]
6%|██ | 2.88M/51.1M [00:02<00:40, 1.18MB/s]
6%|██▏ | 3.01M/51.1M [00:02<00:40, 1.19MB/s]
6%|██▎ | 3.15M/51.1M [00:02<00:40, 1.19MB/s]
6%|██▎ | 3.28M/51.1M [00:02<00:40, 1.19MB/s]
7%|██▍ | 3.41M/51.1M [00:02<00:39, 1.19MB/s]
7%|██▌ | 3.53M/51.1M [00:03<00:39, 1.19MB/s]
7%|██▋ | 3.65M/51.1M [00:03<00:40, 1.18MB/s]
7%|██▋ | 3.78M/51.1M [00:03<00:40, 1.17MB/s]
8%|██▊ | 3.92M/51.1M [00:03<00:40, 1.17MB/s]
8%|██▉ | 4.03M/51.1M [00:03<00:40, 1.16MB/s]
8%|███ | 4.16M/51.1M [00:03<00:40, 1.15MB/s]
8%|███ | 4.29M/51.1M [00:03<00:40, 1.16MB/s]
9%|███▏ | 4.41M/51.1M [00:03<00:40, 1.16MB/s]
9%|███▎ | 4.54M/51.1M [00:03<00:39, 1.18MB/s]
9%|███▎ | 4.66M/51.1M [00:04<00:39, 1.17MB/s]
9%|███▍ | 4.78M/51.1M [00:04<00:39, 1.17MB/s]
10%|███▌ | 4.92M/51.1M [00:04<00:38, 1.19MB/s]
10%|███▋ | 5.04M/51.1M [00:04<00:38, 1.19MB/s]
10%|███▋ | 5.16M/51.1M [00:04<00:39, 1.17MB/s]
10%|███▊ | 5.29M/51.1M [00:04<00:39, 1.17MB/s]
11%|███▉ | 5.42M/51.1M [00:04<00:39, 1.17MB/s]
11%|████ | 5.55M/51.1M [00:04<00:38, 1.17MB/s]
11%|████ | 5.69M/51.1M [00:04<00:38, 1.17MB/s]
11%|████▏ | 5.80M/51.1M [00:05<00:38, 1.16MB/s]
12%|████▎ | 5.93M/51.1M [00:05<00:39, 1.16MB/s]
12%|████▍ | 6.05M/51.1M [00:05<00:39, 1.15MB/s]
12%|████▍ | 6.16M/51.1M [00:05<00:39, 1.14MB/s]
12%|████▌ | 6.29M/51.1M [00:05<00:38, 1.16MB/s]
13%|████▋ | 6.42M/51.1M [00:05<00:37, 1.18MB/s]
13%|████▋ | 6.55M/51.1M [00:05<00:36, 1.21MB/s]
13%|████▊ | 6.68M/51.1M [00:05<00:36, 1.21MB/s]
13%|████▉ | 6.82M/51.1M [00:05<00:37, 1.19MB/s]
14%|█████ | 6.95M/51.1M [00:05<00:37, 1.19MB/s]
14%|█████ | 7.07M/51.1M [00:06<00:37, 1.17MB/s]
14%|█████▏ | 7.18M/51.1M [00:06<00:37, 1.17MB/s]
14%|█████▎ | 7.31M/51.1M [00:06<00:38, 1.14MB/s]
15%|█████▍ | 7.44M/51.1M [00:06<00:37, 1.16MB/s]
15%|█████▍ | 7.57M/51.1M [00:06<00:37, 1.17MB/s]
15%|█████▌ | 7.70M/51.1M [00:06<00:36, 1.18MB/s]
15%|█████▋ | 7.82M/51.1M [00:06<00:36, 1.18MB/s]
16%|█████▊ | 7.95M/51.1M [00:06<00:36, 1.17MB/s]
16%|█████▊ | 8.08M/51.1M [00:06<00:36, 1.17MB/s]
16%|█████▉ | 8.21M/51.1M [00:07<00:36, 1.17MB/s]
16%|██████ | 8.33M/51.1M [00:07<00:36, 1.17MB/s]
17%|██████ | 8.45M/51.1M [00:07<00:36, 1.17MB/s]
17%|██████▏ | 8.59M/51.1M [00:07<00:36, 1.17MB/s]
17%|██████▎ | 8.72M/51.1M [00:07<00:36, 1.18MB/s]
17%|██████▍ | 8.85M/51.1M [00:07<00:36, 1.17MB/s]
18%|██████▍ | 8.97M/51.1M [00:07<00:36, 1.16MB/s]
18%|██████▌ | 9.09M/51.1M [00:07<00:36, 1.16MB/s]
18%|██████▋ | 9.22M/51.1M [00:07<00:36, 1.16MB/s]
18%|██████▊ | 9.36M/51.1M [00:08<00:35, 1.16MB/s]
19%|██████▊ | 9.49M/51.1M [00:08<00:35, 1.16MB/s]
19%|██████▉ | 9.62M/51.1M [00:08<00:35, 1.17MB/s]
19%|███████ | 9.75M/51.1M [00:08<00:35, 1.18MB/s]
19%|███████▏ | 9.87M/51.1M [00:08<00:35, 1.17MB/s]
20%|███████▏ | 9.99M/51.1M [00:08<00:35, 1.16MB/s]
20%|███████▎ | 10.1M/51.1M [00:08<00:35, 1.17MB/s]
20%|███████▍ | 10.3M/51.1M [00:08<00:34, 1.18MB/s]
20%|███████▌ | 10.4M/51.1M [00:08<00:34, 1.19MB/s]
21%|███████▌ | 10.5M/51.1M [00:09<00:34, 1.19MB/s]
21%|███████▋ | 10.6M/51.1M [00:09<00:34, 1.19MB/s]
21%|███████▊ | 10.8M/51.1M [00:09<00:33, 1.20MB/s]
21%|███████▉ | 10.9M/51.1M [00:09<00:33, 1.20MB/s]
22%|███████▉ | 11.0M/51.1M [00:09<00:33, 1.20MB/s]
22%|████████ | 11.1M/51.1M [00:09<00:34, 1.17MB/s]
22%|████████▏ | 11.3M/51.1M [00:09<00:34, 1.17MB/s]
22%|████████▏ | 11.4M/51.1M [00:09<00:33, 1.17MB/s]
23%|████████▎ | 11.5M/51.1M [00:09<00:34, 1.14MB/s]
23%|████████▍ | 11.6M/51.1M [00:09<00:34, 1.15MB/s]
23%|████████▌ | 11.8M/51.1M [00:10<00:34, 1.15MB/s]
23%|████████▌ | 11.9M/51.1M [00:10<00:34, 1.15MB/s]
23%|████████▋ | 12.0M/51.1M [00:10<00:33, 1.15MB/s]
24%|████████▊ | 12.1M/51.1M [00:10<00:34, 1.14MB/s]
24%|████████▊ | 12.2M/51.1M [00:10<00:34, 1.13MB/s]
24%|████████▉ | 12.3M/51.1M [00:10<00:34, 1.13MB/s]
24%|█████████ | 12.5M/51.1M [00:10<00:34, 1.12MB/s]
25%|█████████ | 12.6M/51.1M [00:10<00:34, 1.11MB/s]
25%|█████████▏ | 12.7M/51.1M [00:10<00:34, 1.11MB/s]
25%|█████████▎ | 12.8M/51.1M [00:11<00:33, 1.13MB/s]
25%|█████████▎ | 12.9M/51.1M [00:11<00:33, 1.13MB/s]
26%|█████████▍ | 13.0M/51.1M [00:11<00:33, 1.12MB/s]
26%|█████████▌ | 13.2M/51.1M [00:11<00:33, 1.13MB/s]
26%|█████████▌ | 13.3M/51.1M [00:11<00:33, 1.12MB/s]
26%|█████████▋ | 13.4M/51.1M [00:11<00:33, 1.11MB/s]
26%|█████████▊ | 13.5M/51.1M [00:11<00:33, 1.12MB/s]
27%|█████████▊ | 13.6M/51.1M [00:11<00:33, 1.12MB/s]
27%|█████████▉ | 13.7M/51.1M [00:11<00:33, 1.13MB/s]
27%|██████████ | 13.9M/51.1M [00:11<00:32, 1.14MB/s]
27%|██████████▏ | 14.0M/51.1M [00:12<00:32, 1.13MB/s]
28%|██████████▏ | 14.1M/51.1M [00:12<00:32, 1.14MB/s]
28%|██████████▎ | 14.2M/51.1M [00:12<00:32, 1.14MB/s]
28%|██████████▍ | 14.4M/51.1M [00:12<00:32, 1.13MB/s]
28%|██████████▍ | 14.5M/51.1M [00:12<00:31, 1.15MB/s]
29%|██████████▌ | 14.6M/51.1M [00:12<00:31, 1.15MB/s]
29%|██████████▋ | 14.7M/51.1M [00:12<00:31, 1.15MB/s]
29%|██████████▊ | 14.9M/51.1M [00:12<00:31, 1.16MB/s]
29%|██████████▊ | 15.0M/51.1M [00:12<00:31, 1.16MB/s]
30%|██████████▉ | 15.1M/51.1M [00:13<00:30, 1.17MB/s]
30%|███████████ | 15.2M/51.1M [00:13<00:30, 1.17MB/s]
30%|███████████ | 15.4M/51.1M [00:13<00:30, 1.17MB/s]
30%|███████████▏ | 15.5M/51.1M [00:13<00:30, 1.16MB/s]
31%|███████████▎ | 15.6M/51.1M [00:13<00:30, 1.15MB/s]
31%|███████████▍ | 15.7M/51.1M [00:13<00:30, 1.15MB/s]
31%|███████████▍ | 15.8M/51.1M [00:13<00:30, 1.15MB/s]
31%|███████████▌ | 16.0M/51.1M [00:13<00:30, 1.16MB/s]
31%|███████████▋ | 16.1M/51.1M [00:13<00:30, 1.16MB/s]
32%|███████████▋ | 16.2M/51.1M [00:14<00:29, 1.17MB/s]
32%|███████████▊ | 16.3M/51.1M [00:14<00:30, 1.16MB/s]
32%|███████████▉ | 16.5M/51.1M [00:14<00:30, 1.15MB/s]
32%|████████████ | 16.6M/51.1M [00:14<00:29, 1.17MB/s]
33%|████████████ | 16.7M/51.1M [00:14<00:28, 1.20MB/s]
33%|████████████▏ | 16.9M/51.1M [00:14<00:28, 1.21MB/s]
33%|████████████▎ | 17.0M/51.1M [00:14<00:28, 1.21MB/s]
33%|████████████▍ | 17.1M/51.1M [00:14<00:28, 1.21MB/s]
34%|████████████▍ | 17.3M/51.1M [00:14<00:28, 1.20MB/s]
34%|████████████▌ | 17.4M/51.1M [00:14<00:28, 1.20MB/s]
34%|████████████▋ | 17.5M/51.1M [00:15<00:28, 1.19MB/s]
35%|████████████▊ | 17.6M/51.1M [00:15<00:27, 1.21MB/s]
35%|████████████▊ | 17.8M/51.1M [00:15<00:27, 1.21MB/s]
35%|████████████▉ | 17.9M/51.1M [00:15<00:27, 1.21MB/s]
35%|█████████████ | 18.0M/51.1M [00:15<00:27, 1.20MB/s]
36%|█████████████▏ | 18.2M/51.1M [00:15<00:27, 1.19MB/s]
36%|█████████████▏ | 18.3M/51.1M [00:15<00:27, 1.19MB/s]
36%|█████████████▎ | 18.4M/51.1M [00:15<00:27, 1.18MB/s]
36%|█████████████▍ | 18.6M/51.1M [00:15<00:27, 1.19MB/s]
37%|█████████████▌ | 18.7M/51.1M [00:16<00:27, 1.20MB/s]
37%|█████████████▋ | 18.8M/51.1M [00:16<00:26, 1.20MB/s]
37%|█████████████▋ | 19.0M/51.1M [00:16<00:26, 1.20MB/s]
37%|█████████████▊ | 19.1M/51.1M [00:16<00:26, 1.21MB/s]
38%|█████████████▉ | 19.2M/51.1M [00:16<00:26, 1.23MB/s]
38%|██████████████ | 19.4M/51.1M [00:16<00:25, 1.26MB/s]
38%|██████████████ | 19.5M/51.1M [00:16<00:25, 1.26MB/s]
38%|██████████████▏ | 19.6M/51.1M [00:16<00:25, 1.25MB/s]
39%|██████████████▎ | 19.8M/51.1M [00:16<00:24, 1.28MB/s]
39%|██████████████▍ | 19.9M/51.1M [00:17<00:24, 1.27MB/s]
39%|██████████████▌ | 20.0M/51.1M [00:17<00:24, 1.25MB/s]
39%|██████████████▌ | 20.2M/51.1M [00:17<00:24, 1.27MB/s]
40%|██████████████▋ | 20.3M/51.1M [00:17<00:24, 1.24MB/s]
40%|██████████████▊ | 20.4M/51.1M [00:17<00:24, 1.25MB/s]
40%|██████████████▉ | 20.6M/51.1M [00:17<00:24, 1.25MB/s]
40%|██████████████▉ | 20.7M/51.1M [00:17<00:24, 1.23MB/s]
41%|███████████████ | 20.8M/51.1M [00:17<00:24, 1.23MB/s]
41%|███████████████▏ | 21.0M/51.1M [00:17<00:24, 1.23MB/s]
41%|███████████████▎ | 21.1M/51.1M [00:17<00:24, 1.24MB/s]
42%|███████████████▎ | 21.2M/51.1M [00:18<00:24, 1.24MB/s]
42%|███████████████▍ | 21.3M/51.1M [00:18<00:23, 1.24MB/s]
42%|███████████████▌ | 21.5M/51.1M [00:18<00:23, 1.25MB/s]
42%|███████████████▋ | 21.6M/51.1M [00:18<00:23, 1.24MB/s]
43%|███████████████▋ | 21.7M/51.1M [00:18<00:23, 1.23MB/s]
43%|███████████████▊ | 21.9M/51.1M [00:18<00:24, 1.21MB/s]
43%|███████████████▉ | 22.0M/51.1M [00:18<00:23, 1.22MB/s]
43%|████████████████ | 22.1M/51.1M [00:18<00:23, 1.24MB/s]
44%|████████████████ | 22.3M/51.1M [00:18<00:23, 1.25MB/s]
44%|████████████████▏ | 22.4M/51.1M [00:19<00:22, 1.26MB/s]
44%|████████████████▎ | 22.5M/51.1M [00:19<00:22, 1.26MB/s]
44%|████████████████▍ | 22.7M/51.1M [00:19<00:23, 1.23MB/s]
45%|████████████████▍ | 22.8M/51.1M [00:19<00:23, 1.20MB/s]
45%|████████████████▌ | 22.9M/51.1M [00:19<00:23, 1.21MB/s]
45%|████████████████▋ | 23.1M/51.1M [00:19<00:23, 1.21MB/s]
45%|████████████████▊ | 23.2M/51.1M [00:19<00:23, 1.21MB/s]
46%|████████████████▉ | 23.3M/51.1M [00:19<00:23, 1.19MB/s]
46%|████████████████▉ | 23.4M/51.1M [00:19<00:23, 1.20MB/s]
46%|█████████████████ | 23.6M/51.1M [00:20<00:22, 1.20MB/s]
46%|█████████████████▏ | 23.7M/51.1M [00:20<00:22, 1.21MB/s]
47%|█████████████████▎ | 23.8M/51.1M [00:20<00:22, 1.20MB/s]
47%|█████████████████▎ | 24.0M/51.1M [00:20<00:22, 1.18MB/s]
47%|█████████████████▍ | 24.1M/51.1M [00:20<00:22, 1.19MB/s]
47%|█████████████████▌ | 24.2M/51.1M [00:20<00:22, 1.18MB/s]
48%|█████████████████▋ | 24.3M/51.1M [00:20<00:22, 1.18MB/s]
48%|█████████████████▋ | 24.5M/51.1M [00:20<00:22, 1.18MB/s]
48%|█████████████████▊ | 24.6M/51.1M [00:20<00:22, 1.18MB/s]
48%|█████████████████▉ | 24.7M/51.1M [00:21<00:22, 1.18MB/s]
49%|██████████████████ | 24.9M/51.1M [00:21<00:22, 1.18MB/s]
49%|██████████████████ | 25.0M/51.1M [00:21<00:22, 1.18MB/s]
49%|██████████████████▏ | 25.1M/51.1M [00:21<00:21, 1.21MB/s]
49%|██████████████████▎ | 25.3M/51.1M [00:21<00:21, 1.21MB/s]
50%|██████████████████▍ | 25.4M/51.1M [00:21<00:21, 1.22MB/s]
50%|██████████████████▍ | 25.5M/51.1M [00:21<00:21, 1.20MB/s]
50%|██████████████████▌ | 25.7M/51.1M [00:21<00:21, 1.20MB/s]
50%|██████████████████▋ | 25.8M/51.1M [00:21<00:21, 1.19MB/s]
51%|██████████████████▊ | 25.9M/51.1M [00:21<00:21, 1.20MB/s]
51%|██████████████████▊ | 26.1M/51.1M [00:22<00:20, 1.21MB/s]
51%|██████████████████▉ | 26.2M/51.1M [00:22<00:20, 1.21MB/s]
51%|███████████████████ | 26.3M/51.1M [00:22<00:20, 1.19MB/s]
52%|███████████████████▏ | 26.4M/51.1M [00:22<00:20, 1.19MB/s]
52%|███████████████████▏ | 26.6M/51.1M [00:22<00:20, 1.19MB/s]
52%|███████████████████▎ | 26.7M/51.1M [00:22<00:20, 1.18MB/s]
52%|███████████████████▍ | 26.8M/51.1M [00:22<00:20, 1.16MB/s]
53%|███████████████████▍ | 26.9M/51.1M [00:22<00:20, 1.17MB/s]
53%|███████████████████▌ | 27.1M/51.1M [00:22<00:20, 1.17MB/s]
53%|███████████████████▋ | 27.2M/51.1M [00:23<00:20, 1.18MB/s]
53%|███████████████████▊ | 27.3M/51.1M [00:23<00:20, 1.18MB/s]
54%|███████████████████▊ | 27.4M/51.1M [00:23<00:19, 1.20MB/s]
54%|███████████████████▉ | 27.6M/51.1M [00:23<00:19, 1.22MB/s]
54%|████████████████████ | 27.7M/51.1M [00:23<00:19, 1.20MB/s]
54%|████████████████████▏ | 27.8M/51.1M [00:23<00:19, 1.21MB/s]
55%|████████████████████▏ | 28.0M/51.1M [00:23<00:19, 1.21MB/s]
55%|████████████████████▎ | 28.1M/51.1M [00:23<00:19, 1.20MB/s]
55%|████████████████████▍ | 28.2M/51.1M [00:23<00:19, 1.20MB/s]
55%|████████████████████▌ | 28.4M/51.1M [00:24<00:19, 1.20MB/s]
56%|████████████████████▋ | 28.5M/51.1M [00:24<00:18, 1.20MB/s]
56%|████████████████████▋ | 28.6M/51.1M [00:24<00:18, 1.21MB/s]
56%|████████████████████▊ | 28.7M/51.1M [00:24<00:18, 1.21MB/s]
56%|████████████████████▉ | 28.9M/51.1M [00:24<00:18, 1.18MB/s]
57%|████████████████████▉ | 29.0M/51.1M [00:24<00:18, 1.19MB/s]
57%|█████████████████████ | 29.1M/51.1M [00:24<00:18, 1.19MB/s]
57%|█████████████████████▏ | 29.3M/51.1M [00:24<00:18, 1.19MB/s]
58%|█████████████████████▎ | 29.4M/51.1M [00:24<00:18, 1.19MB/s]
58%|█████████████████████▎ | 29.5M/51.1M [00:25<00:18, 1.19MB/s]
58%|█████████████████████▍ | 29.7M/51.1M [00:25<00:18, 1.19MB/s]
58%|█████████████████████▌ | 29.8M/51.1M [00:25<00:18, 1.18MB/s]
59%|█████████████████████▋ | 29.9M/51.1M [00:25<00:17, 1.19MB/s]
59%|█████████████████████▋ | 30.0M/51.1M [00:25<00:17, 1.19MB/s]
59%|█████████████████████▊ | 30.2M/51.1M [00:25<00:17, 1.19MB/s]
59%|█████████████████████▉ | 30.3M/51.1M [00:25<00:17, 1.20MB/s]
60%|██████████████████████ | 30.4M/51.1M [00:25<00:17, 1.20MB/s]
60%|██████████████████████ | 30.6M/51.1M [00:25<00:17, 1.21MB/s]
60%|██████████████████████▏ | 30.7M/51.1M [00:25<00:17, 1.19MB/s]
60%|██████████████████████▎ | 30.8M/51.1M [00:26<00:17, 1.18MB/s]
61%|██████████████████████▍ | 30.9M/51.1M [00:26<00:16, 1.20MB/s]
61%|██████████████████████▍ | 31.1M/51.1M [00:26<00:16, 1.21MB/s]
61%|██████████████████████▌ | 31.2M/51.1M [00:26<00:16, 1.22MB/s]
61%|██████████████████████▋ | 31.3M/51.1M [00:26<00:16, 1.21MB/s]
62%|██████████████████████▊ | 31.5M/51.1M [00:26<00:16, 1.21MB/s]
62%|██████████████████████▉ | 31.6M/51.1M [00:26<00:16, 1.20MB/s]
62%|██████████████████████▉ | 31.7M/51.1M [00:26<00:16, 1.20MB/s]
62%|███████████████████████ | 31.9M/51.1M [00:26<00:16, 1.19MB/s]
63%|███████████████████████▏ | 32.0M/51.1M [00:27<00:16, 1.19MB/s]
63%|███████████████████████▏ | 32.1M/51.1M [00:27<00:15, 1.21MB/s]
63%|███████████████████████▎ | 32.2M/51.1M [00:27<00:15, 1.21MB/s]
63%|███████████████████████▍ | 32.4M/51.1M [00:27<00:15, 1.22MB/s]
64%|███████████████████████▌ | 32.5M/51.1M [00:27<00:15, 1.23MB/s]
64%|███████████████████████▋ | 32.6M/51.1M [00:27<00:15, 1.22MB/s]
64%|███████████████████████▋ | 32.8M/51.1M [00:27<00:15, 1.21MB/s]
64%|███████████████████████▊ | 32.9M/51.1M [00:27<00:15, 1.21MB/s]
65%|███████████████████████▉ | 33.0M/51.1M [00:27<00:15, 1.20MB/s]
65%|████████████████████████ | 33.2M/51.1M [00:28<00:15, 1.19MB/s]
65%|████████████████████████ | 33.3M/51.1M [00:28<00:15, 1.19MB/s]
65%|████████████████████████▏ | 33.4M/51.1M [00:28<00:14, 1.19MB/s]
66%|████████████████████████▎ | 33.6M/51.1M [00:28<00:14, 1.18MB/s]
66%|████████████████████████▍ | 33.7M/51.1M [00:28<00:14, 1.18MB/s]
66%|████████████████████████▍ | 33.8M/51.1M [00:28<00:14, 1.18MB/s]
66%|████████████████████████▌ | 33.9M/51.1M [00:28<00:14, 1.18MB/s]
67%|████████████████████████▋ | 34.1M/51.1M [00:28<00:14, 1.18MB/s]
67%|████████████████████████▋ | 34.2M/51.1M [00:28<00:14, 1.19MB/s]
67%|████████████████████████▊ | 34.3M/51.1M [00:29<00:14, 1.18MB/s]
67%|████████████████████████▉ | 34.4M/51.1M [00:29<00:14, 1.17MB/s]
68%|█████████████████████████ | 34.6M/51.1M [00:29<00:13, 1.19MB/s]
68%|█████████████████████████ | 34.7M/51.1M [00:29<00:13, 1.19MB/s]
68%|█████████████████████████▏ | 34.8M/51.1M [00:29<00:13, 1.19MB/s]
68%|█████████████████████████▎ | 34.9M/51.1M [00:29<00:13, 1.18MB/s]
69%|█████████████████████████▍ | 35.1M/51.1M [00:29<00:13, 1.17MB/s]
69%|█████████████████████████▍ | 35.2M/51.1M [00:29<00:13, 1.17MB/s]
69%|█████████████████████████▌ | 35.3M/51.1M [00:29<00:13, 1.17MB/s]
69%|█████████████████████████▋ | 35.5M/51.1M [00:29<00:13, 1.18MB/s]
70%|█████████████████████████▊ | 35.6M/51.1M [00:30<00:13, 1.18MB/s]
70%|█████████████████████████▊ | 35.7M/51.1M [00:30<00:13, 1.18MB/s]
70%|█████████████████████████▉ | 35.8M/51.1M [00:30<00:12, 1.19MB/s]
70%|██████████████████████████ | 36.0M/51.1M [00:30<00:12, 1.19MB/s]
71%|██████████████████████████▏ | 36.1M/51.1M [00:30<00:12, 1.19MB/s]
71%|██████████████████████████▏ | 36.2M/51.1M [00:30<00:12, 1.20MB/s]
71%|██████████████████████████▎ | 36.4M/51.1M [00:30<00:12, 1.19MB/s]
71%|██████████████████████████▍ | 36.5M/51.1M [00:30<00:12, 1.18MB/s]
72%|██████████████████████████▌ | 36.6M/51.1M [00:30<00:12, 1.18MB/s]
72%|██████████████████████████▌ | 36.7M/51.1M [00:31<00:12, 1.18MB/s]
72%|██████████████████████████▋ | 36.9M/51.1M [00:31<00:12, 1.18MB/s]
72%|██████████████████████████▊ | 37.0M/51.1M [00:31<00:11, 1.22MB/s]
73%|██████████████████████████▉ | 37.1M/51.1M [00:31<00:11, 1.22MB/s]
73%|██████████████████████████▉ | 37.3M/51.1M [00:31<00:11, 1.22MB/s]
73%|███████████████████████████ | 37.4M/51.1M [00:31<00:11, 1.23MB/s]
73%|███████████████████████████▏ | 37.5M/51.1M [00:31<00:10, 1.24MB/s]
74%|███████████████████████████▎ | 37.7M/51.1M [00:31<00:11, 1.22MB/s]
74%|███████████████████████████▎ | 37.8M/51.1M [00:31<00:10, 1.22MB/s]
74%|███████████████████████████▍ | 37.9M/51.1M [00:32<00:10, 1.23MB/s]
74%|███████████████████████████▌ | 38.1M/51.1M [00:32<00:10, 1.25MB/s]
75%|███████████████████████████▋ | 38.2M/51.1M [00:32<00:10, 1.26MB/s]
75%|███████████████████████████▊ | 38.3M/51.1M [00:32<00:09, 1.28MB/s]
75%|███████████████████████████▊ | 38.5M/51.1M [00:32<00:09, 1.29MB/s]
76%|███████████████████████████▉ | 38.6M/51.1M [00:32<00:09, 1.29MB/s]
76%|████████████████████████████ | 38.7M/51.1M [00:32<00:09, 1.27MB/s]
76%|████████████████████████████▏ | 38.9M/51.1M [00:32<00:09, 1.27MB/s]
76%|████████████████████████████▏ | 39.0M/51.1M [00:32<00:09, 1.26MB/s]
77%|████████████████████████████▎ | 39.1M/51.1M [00:32<00:09, 1.26MB/s]
77%|████████████████████████████▍ | 39.3M/51.1M [00:33<00:09, 1.26MB/s]
77%|████████████████████████████▌ | 39.4M/51.1M [00:33<00:09, 1.26MB/s]
77%|████████████████████████████▌ | 39.5M/51.1M [00:33<00:09, 1.26MB/s]
78%|████████████████████████████▋ | 39.7M/51.1M [00:33<00:09, 1.25MB/s]
78%|████████████████████████████▊ | 39.8M/51.1M [00:33<00:09, 1.24MB/s]
78%|████████████████████████████▉ | 39.9M/51.1M [00:33<00:08, 1.25MB/s]
78%|████████████████████████████▉ | 40.1M/51.1M [00:33<00:09, 1.22MB/s]
79%|█████████████████████████████ | 40.2M/51.1M [00:33<00:08, 1.22MB/s]
79%|█████████████████████████████▏ | 40.3M/51.1M [00:33<00:08, 1.21MB/s]
79%|█████████████████████████████▎ | 40.5M/51.1M [00:34<00:08, 1.20MB/s]
79%|█████████████████████████████▍ | 40.6M/51.1M [00:34<00:08, 1.20MB/s]
80%|█████████████████████████████▍ | 40.7M/51.1M [00:34<00:08, 1.20MB/s]
80%|█████████████████████████████▌ | 40.8M/51.1M [00:34<00:08, 1.21MB/s]
80%|█████████████████████████████▋ | 41.0M/51.1M [00:34<00:08, 1.20MB/s]
80%|█████████████████████████████▊ | 41.1M/51.1M [00:34<00:08, 1.21MB/s]
81%|█████████████████████████████▊ | 41.2M/51.1M [00:34<00:08, 1.21MB/s]
81%|█████████████████████████████▉ | 41.4M/51.1M [00:34<00:08, 1.21MB/s]
81%|██████████████████████████████ | 41.5M/51.1M [00:34<00:07, 1.23MB/s]
81%|██████████████████████████████▏ | 41.6M/51.1M [00:35<00:07, 1.23MB/s]
82%|██████████████████████████████▏ | 41.8M/51.1M [00:35<00:07, 1.22MB/s]
82%|██████████████████████████████▎ | 41.9M/51.1M [00:35<00:07, 1.22MB/s]
82%|██████████████████████████████▍ | 42.0M/51.1M [00:35<00:07, 1.23MB/s]
82%|██████████████████████████████▌ | 42.2M/51.1M [00:35<00:07, 1.22MB/s]
83%|██████████████████████████████▌ | 42.3M/51.1M [00:35<00:07, 1.23MB/s]
83%|██████████████████████████████▋ | 42.4M/51.1M [00:35<00:07, 1.22MB/s]
83%|██████████████████████████████▊ | 42.5M/51.1M [00:35<00:07, 1.20MB/s]
84%|██████████████████████████████▉ | 42.7M/51.1M [00:35<00:07, 1.20MB/s]
84%|██████████████████████████████▉ | 42.8M/51.1M [00:36<00:06, 1.19MB/s]
84%|███████████████████████████████ | 42.9M/51.1M [00:36<00:06, 1.20MB/s]
84%|███████████████████████████████▏ | 43.1M/51.1M [00:36<00:06, 1.21MB/s]
85%|███████████████████████████████▎ | 43.2M/51.1M [00:36<00:06, 1.21MB/s]
85%|███████████████████████████████▎ | 43.3M/51.1M [00:36<00:06, 1.21MB/s]
85%|███████████████████████████████▍ | 43.5M/51.1M [00:36<00:06, 1.25MB/s]
85%|███████████████████████████████▌ | 43.6M/51.1M [00:36<00:06, 1.24MB/s]
86%|███████████████████████████████▋ | 43.7M/51.1M [00:36<00:06, 1.22MB/s]
86%|███████████████████████████████▊ | 43.9M/51.1M [00:36<00:05, 1.21MB/s]
86%|███████████████████████████████▊ | 44.0M/51.1M [00:36<00:05, 1.20MB/s]
86%|███████████████████████████████▉ | 44.1M/51.1M [00:37<00:05, 1.19MB/s]
87%|████████████████████████████████ | 44.3M/51.1M [00:37<00:05, 1.17MB/s]
87%|████████████████████████████████▏ | 44.4M/51.1M [00:37<00:05, 1.18MB/s]
87%|████████████████████████████████▏ | 44.5M/51.1M [00:37<00:05, 1.19MB/s]
87%|████████████████████████████████▎ | 44.7M/51.1M [00:37<00:05, 1.19MB/s]
88%|████████████████████████████████▍ | 44.8M/51.1M [00:37<00:05, 1.18MB/s]
88%|████████████████████████████████▌ | 44.9M/51.1M [00:37<00:05, 1.17MB/s]
88%|████████████████████████████████▌ | 45.0M/51.1M [00:37<00:05, 1.16MB/s]
88%|████████████████████████████████▋ | 45.1M/51.1M [00:37<00:05, 1.14MB/s]
89%|████████████████████████████████▊ | 45.3M/51.1M [00:38<00:05, 1.15MB/s]
89%|████████████████████████████████▊ | 45.4M/51.1M [00:38<00:04, 1.17MB/s]
89%|████████████████████████████████▉ | 45.5M/51.1M [00:38<00:04, 1.17MB/s]
89%|█████████████████████████████████ | 45.6M/51.1M [00:38<00:04, 1.16MB/s]
90%|█████████████████████████████████ | 45.8M/51.1M [00:38<00:04, 1.15MB/s]
90%|█████████████████████████████████▏ | 45.9M/51.1M [00:38<00:04, 1.14MB/s]
90%|█████████████████████████████████▎ | 46.0M/51.1M [00:38<00:04, 1.14MB/s]
90%|█████████████████████████████████▍ | 46.1M/51.1M [00:38<00:04, 1.14MB/s]
90%|█████████████████████████████████▍ | 46.2M/51.1M [00:38<00:04, 1.13MB/s]
91%|█████████████████████████████████▌ | 46.3M/51.1M [00:39<00:04, 1.13MB/s]
91%|█████████████████████████████████▋ | 46.4M/51.1M [00:39<00:04, 1.13MB/s]
91%|█████████████████████████████████▋ | 46.6M/51.1M [00:39<00:04, 1.12MB/s]
91%|█████████████████████████████████▊ | 46.7M/51.1M [00:39<00:03, 1.12MB/s]
92%|█████████████████████████████████▊ | 46.8M/51.1M [00:39<00:03, 1.13MB/s]
92%|█████████████████████████████████▉ | 46.9M/51.1M [00:39<00:03, 1.13MB/s]
92%|██████████████████████████████████ | 47.0M/51.1M [00:39<00:03, 1.15MB/s]
92%|██████████████████████████████████▏ | 47.2M/51.1M [00:39<00:03, 1.14MB/s]
93%|██████████████████████████████████▏ | 47.3M/51.1M [00:39<00:03, 1.17MB/s]
93%|██████████████████████████████████▎ | 47.4M/51.1M [00:39<00:03, 1.17MB/s]
93%|██████████████████████████████████▍ | 47.5M/51.1M [00:40<00:03, 1.18MB/s]
93%|██████████████████████████████████▌ | 47.7M/51.1M [00:40<00:02, 1.18MB/s]
94%|██████████████████████████████████▌ | 47.8M/51.1M [00:40<00:02, 1.18MB/s]
94%|██████████████████████████████████▋ | 47.9M/51.1M [00:40<00:02, 1.16MB/s]
94%|██████████████████████████████████▊ | 48.0M/51.1M [00:40<00:02, 1.15MB/s]
94%|██████████████████████████████████▊ | 48.1M/51.1M [00:40<00:02, 1.13MB/s]
94%|██████████████████████████████████▉ | 48.3M/51.1M [00:40<00:02, 1.12MB/s]
95%|███████████████████████████████████ | 48.4M/51.1M [00:40<00:02, 1.11MB/s]
95%|███████████████████████████████████ | 48.5M/51.1M [00:40<00:02, 1.10MB/s]
95%|███████████████████████████████████▏ | 48.6M/51.1M [00:41<00:02, 1.09MB/s]
95%|███████████████████████████████████▎ | 48.7M/51.1M [00:41<00:02, 1.10MB/s]
96%|███████████████████████████████████▎ | 48.8M/51.1M [00:41<00:02, 1.12MB/s]
96%|███████████████████████████████████▍ | 49.0M/51.1M [00:41<00:01, 1.13MB/s]
96%|███████████████████████████████████▌ | 49.1M/51.1M [00:41<00:01, 1.12MB/s]
96%|███████████████████████████████████▌ | 49.2M/51.1M [00:41<00:01, 1.12MB/s]
96%|███████████████████████████████████▋ | 49.3M/51.1M [00:41<00:01, 1.14MB/s]
97%|███████████████████████████████████▊ | 49.4M/51.1M [00:41<00:01, 1.14MB/s]
97%|███████████████████████████████████▉ | 49.6M/51.1M [00:41<00:01, 1.15MB/s]
97%|███████████████████████████████████▉ | 49.7M/51.1M [00:41<00:01, 1.13MB/s]
97%|████████████████████████████████████ | 49.8M/51.1M [00:42<00:01, 1.13MB/s]
98%|████████████████████████████████████▏| 49.9M/51.1M [00:42<00:01, 1.13MB/s]
98%|████████████████████████████████████▏| 50.0M/51.1M [00:42<00:00, 1.13MB/s]
98%|████████████████████████████████████▎| 50.1M/51.1M [00:42<00:00, 1.12MB/s]
98%|████████████████████████████████████▍| 50.2M/51.1M [00:42<00:00, 1.11MB/s]
99%|████████████████████████████████████▍| 50.4M/51.1M [00:42<00:00, 1.12MB/s]
99%|████████████████████████████████████▌| 50.5M/51.1M [00:42<00:00, 1.11MB/s]
99%|████████████████████████████████████▋| 50.6M/51.1M [00:42<00:00, 1.11MB/s]
99%|████████████████████████████████████▋| 50.7M/51.1M [00:42<00:00, 1.13MB/s]
99%|████████████████████████████████████▊| 50.8M/51.1M [00:42<00:00, 1.12MB/s]
100%|████████████████████████████████████▉| 51.0M/51.1M [00:43<00:00, 1.13MB/s]
100%|████████████████████████████████████▉| 51.1M/51.1M [00:43<00:00, 1.14MB/s]
0%| | 0.00/51.1M [00:00<?, ?B/s]
100%|██████████████████████████████████████| 51.1M/51.1M [00:00<00:00, 326GB/s]
0%| | 0.00/3.90k [00:00<?, ?B/s]
0%| | 0.00/3.90k [00:00<?, ?B/s]
100%|█████████████████████████████████████| 3.90k/3.90k [00:00<00:00, 31.7MB/s]
Download complete in 44s (48.7 MB)
Extracting EDF parameters from /home/runner/mne_data/physionet-sleep-data/SC4001E0-PSG.edf...
EDF file detected
Setting channel info structure...
Creating raw.info structure...
Extracting EDF parameters from /home/runner/mne_data/physionet-sleep-data/SC4011E0-PSG.edf...
EDF file detected
Setting channel info structure...
Creating raw.info structure...
Reading 0 ... 2507000 = 0.000 ... 25070.000 secs...
Filtering raw data in 1 contiguous segment
Setting up low-pass filter at 30 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal lowpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Upper passband edge: 30.00 Hz
- Upper transition bandwidth: 7.50 Hz (-6 dB cutoff frequency: 33.75 Hz)
- Filter length: 45 samples (0.450 s)
Writing /tmp/tmplkulbaqd/0/0-raw.fif
Closing /tmp/tmplkulbaqd/0/0-raw.fif
[done]
Reading 0 ... 3260000 = 0.000 ... 32600.000 secs...
Filtering raw data in 1 contiguous segment
Setting up low-pass filter at 30 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal lowpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Upper passband edge: 30.00 Hz
- Upper transition bandwidth: 7.50 Hz (-6 dB cutoff frequency: 33.75 Hz)
- Filter length: 45 samples (0.450 s)
Writing /tmp/tmplkulbaqd/1/1-raw.fif
Closing /tmp/tmplkulbaqd/1/1-raw.fif
[done]
/home/runner/work/braindecode/braindecode/braindecode/datasets/base.py:708: UserWarning: Chosen directory /tmp/tmplkulbaqd contains other subdirectories or files ['0'].
warnings.warn(f'Chosen directory {path} contains other '
Opening raw data file /tmp/tmplkulbaqd/0/0-raw.fif...
Isotrak not found
Range : 2884000 ... 5391000 = 28840.000 ... 53910.000 secs
Ready.
Opening raw data file /tmp/tmplkulbaqd/1/1-raw.fif...
Isotrak not found
Range : 1975000 ... 5235000 = 19750.000 ... 52350.000 secs
Ready.
Reading 0 ... 2507000 = 0.000 ... 25070.000 secs...
Writing /tmp/tmplkulbaqd/0/0-raw.fif
Closing /tmp/tmplkulbaqd/0/0-raw.fif
[done]
/home/runner/work/braindecode/braindecode/braindecode/datasets/base.py:700: UserWarning: The number of saved datasets (1) does not match the number of existing subdirectories (2). You may now encounter a mix of differently preprocessed datasets!
warnings.warn(f"The number of saved datasets ({i_ds+1+offset}) "
/home/runner/work/braindecode/braindecode/braindecode/datasets/base.py:708: UserWarning: Chosen directory /tmp/tmplkulbaqd contains other subdirectories or files ['1'].
warnings.warn(f'Chosen directory {path} contains other '
Reading 0 ... 3260000 = 0.000 ... 32600.000 secs...
Writing /tmp/tmplkulbaqd/1/1-raw.fif
Closing /tmp/tmplkulbaqd/1/1-raw.fif
[done]
/home/runner/work/braindecode/braindecode/braindecode/datasets/base.py:708: UserWarning: Chosen directory /tmp/tmplkulbaqd contains other subdirectories or files ['0'].
warnings.warn(f'Chosen directory {path} contains other '
Opening raw data file /tmp/tmplkulbaqd/0/0-raw.fif...
Isotrak not found
Range : 2884000 ... 5391000 = 28840.000 ... 53910.000 secs
Ready.
Opening raw data file /tmp/tmplkulbaqd/1/1-raw.fif...
Isotrak not found
Range : 1975000 ... 5235000 = 19750.000 ... 52350.000 secs
Ready.
Extracting EDF parameters from /home/runner/mne_data/physionet-sleep-data/SC4001E0-PSG.edf...
EDF file detected
Setting channel info structure...
Creating raw.info structure...
Extracting EDF parameters from /home/runner/mne_data/physionet-sleep-data/SC4011E0-PSG.edf...
EDF file detected
Setting channel info structure...
Creating raw.info structure...
Opening raw data file /tmp/tmp6e7o1nnr/0/0-raw.fif...
Isotrak not found
Range : 2884000 ... 5391000 = 28840.000 ... 53910.000 secs
Ready.
Opening raw data file /tmp/tmp6e7o1nnr/1/1-raw.fif...
Isotrak not found
Range : 1975000 ... 5235000 = 19750.000 ... 52350.000 secs
Ready.
Opening raw data file /tmp/tmp6e7o1nnr/0/0-raw.fif...
Isotrak not found
Range : 2884000 ... 5391000 = 28840.000 ... 53910.000 secs
Ready.
Opening raw data file /tmp/tmp6e7o1nnr/1/1-raw.fif...
Isotrak not found
Range : 1975000 ... 5235000 = 19750.000 ... 52350.000 secs
Ready.
Extracting EDF parameters from /home/runner/mne_data/physionet-sleep-data/SC4001E0-PSG.edf...
EDF file detected
Setting channel info structure...
Creating raw.info structure...
Extracting EDF parameters from /home/runner/mne_data/physionet-sleep-data/SC4011E0-PSG.edf...
EDF file detected
Setting channel info structure...
Creating raw.info structure...
Reading 0 ... 2507000 = 0.000 ... 25070.000 secs...
Filtering raw data in 1 contiguous segment
Setting up low-pass filter at 30 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal lowpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Upper passband edge: 30.00 Hz
- Upper transition bandwidth: 7.50 Hz (-6 dB cutoff frequency: 33.75 Hz)
- Filter length: 45 samples (0.450 s)
Reading 0 ... 3260000 = 0.000 ... 32600.000 secs...
Filtering raw data in 1 contiguous segment
Setting up low-pass filter at 30 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal lowpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Upper passband edge: 30.00 Hz
- Upper transition bandwidth: 7.50 Hz (-6 dB cutoff frequency: 33.75 Hz)
- Filter length: 45 samples (0.450 s)
Extracting EDF parameters from /home/runner/mne_data/physionet-sleep-data/SC4001E0-PSG.edf...
EDF file detected
Setting channel info structure...
Creating raw.info structure...
Extracting EDF parameters from /home/runner/mne_data/physionet-sleep-data/SC4011E0-PSG.edf...
EDF file detected
Setting channel info structure...
Creating raw.info structure...
Extracting EDF parameters from /home/runner/mne_data/physionet-sleep-data/SC4001E0-PSG.edf...
EDF file detected
Setting channel info structure...
Creating raw.info structure...
Extracting EDF parameters from /home/runner/mne_data/physionet-sleep-data/SC4011E0-PSG.edf...
EDF file detected
Setting channel info structure...
Creating raw.info structure...
Reading 0 ... 2507000 = 0.000 ... 25070.000 secs...
Filtering raw data in 1 contiguous segment
Setting up low-pass filter at 30 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal lowpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Upper passband edge: 30.00 Hz
- Upper transition bandwidth: 7.50 Hz (-6 dB cutoff frequency: 33.75 Hz)
- Filter length: 45 samples (0.450 s)
Writing /tmp/tmppcdjcl1n/0/0-raw.fif
Closing /tmp/tmppcdjcl1n/0/0-raw.fif
[done]
Reading 0 ... 3260000 = 0.000 ... 32600.000 secs...
Filtering raw data in 1 contiguous segment
Setting up low-pass filter at 30 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal lowpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Upper passband edge: 30.00 Hz
- Upper transition bandwidth: 7.50 Hz (-6 dB cutoff frequency: 33.75 Hz)
- Filter length: 45 samples (0.450 s)
Writing /tmp/tmppcdjcl1n/1/1-raw.fif
Closing /tmp/tmppcdjcl1n/1/1-raw.fif
[done]
/home/runner/work/braindecode/braindecode/braindecode/datasets/base.py:708: UserWarning: Chosen directory /tmp/tmppcdjcl1n contains other subdirectories or files ['0'].
warnings.warn(f'Chosen directory {path} contains other '
Opening raw data file /tmp/tmppcdjcl1n/0/0-raw.fif...
Isotrak not found
Range : 2884000 ... 5391000 = 28840.000 ... 53910.000 secs
Ready.
Opening raw data file /tmp/tmppcdjcl1n/1/1-raw.fif...
Isotrak not found
Range : 1975000 ... 5235000 = 19750.000 ... 52350.000 secs
Ready.
Reading 0 ... 2507000 = 0.000 ... 25070.000 secs...
Writing /tmp/tmppcdjcl1n/0/0-raw.fif
Closing /tmp/tmppcdjcl1n/0/0-raw.fif
[done]
/home/runner/work/braindecode/braindecode/braindecode/datasets/base.py:700: UserWarning: The number of saved datasets (1) does not match the number of existing subdirectories (2). You may now encounter a mix of differently preprocessed datasets!
warnings.warn(f"The number of saved datasets ({i_ds+1+offset}) "
/home/runner/work/braindecode/braindecode/braindecode/datasets/base.py:708: UserWarning: Chosen directory /tmp/tmppcdjcl1n contains other subdirectories or files ['1'].
warnings.warn(f'Chosen directory {path} contains other '
Reading 0 ... 3260000 = 0.000 ... 32600.000 secs...
Writing /tmp/tmppcdjcl1n/1/1-raw.fif
Closing /tmp/tmppcdjcl1n/1/1-raw.fif
[done]
/home/runner/work/braindecode/braindecode/braindecode/datasets/base.py:708: UserWarning: Chosen directory /tmp/tmppcdjcl1n contains other subdirectories or files ['0'].
warnings.warn(f'Chosen directory {path} contains other '
Opening raw data file /tmp/tmppcdjcl1n/0/0-raw.fif...
Isotrak not found
Range : 2884000 ... 5391000 = 28840.000 ... 53910.000 secs
Ready.
Opening raw data file /tmp/tmppcdjcl1n/1/1-raw.fif...
Isotrak not found
Range : 1975000 ... 5235000 = 19750.000 ... 52350.000 secs
Ready.
Extracting EDF parameters from /home/runner/mne_data/physionet-sleep-data/SC4001E0-PSG.edf...
EDF file detected
Setting channel info structure...
Creating raw.info structure...
Extracting EDF parameters from /home/runner/mne_data/physionet-sleep-data/SC4011E0-PSG.edf...
EDF file detected
Setting channel info structure...
Creating raw.info structure...
Opening raw data file /tmp/tmpilhgpjxk/0/0-raw.fif...
Isotrak not found
Range : 2884000 ... 5391000 = 28840.000 ... 53910.000 secs
Ready.
Opening raw data file /tmp/tmpilhgpjxk/1/1-raw.fif...
Isotrak not found
Range : 1975000 ... 5235000 = 19750.000 ... 52350.000 secs
Ready.
Opening raw data file /tmp/tmpilhgpjxk/0/0-raw.fif...
Isotrak not found
Range : 2884000 ... 5391000 = 28840.000 ... 53910.000 secs
Ready.
Opening raw data file /tmp/tmpilhgpjxk/1/1-raw.fif...
Isotrak not found
Range : 1975000 ... 5235000 = 19750.000 ... 52350.000 secs
Ready.
Extracting EDF parameters from /home/runner/mne_data/physionet-sleep-data/SC4001E0-PSG.edf...
EDF file detected
Setting channel info structure...
Creating raw.info structure...
Extracting EDF parameters from /home/runner/mne_data/physionet-sleep-data/SC4011E0-PSG.edf...
EDF file detected
Setting channel info structure...
Creating raw.info structure...
Reading 0 ... 2507000 = 0.000 ... 25070.000 secs...
Filtering raw data in 1 contiguous segment
Setting up low-pass filter at 30 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal lowpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Upper passband edge: 30.00 Hz
- Upper transition bandwidth: 7.50 Hz (-6 dB cutoff frequency: 33.75 Hz)
- Filter length: 45 samples (0.450 s)
Reading 0 ... 3260000 = 0.000 ... 32600.000 secs...
Filtering raw data in 1 contiguous segment
Setting up low-pass filter at 30 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal lowpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Upper passband edge: 30.00 Hz
- Upper transition bandwidth: 7.50 Hz (-6 dB cutoff frequency: 33.75 Hz)
- Filter length: 45 samples (0.450 s)
Extracting EDF parameters from /home/runner/mne_data/physionet-sleep-data/SC4001E0-PSG.edf...
EDF file detected
Setting channel info structure...
Creating raw.info structure...
Extracting EDF parameters from /home/runner/mne_data/physionet-sleep-data/SC4011E0-PSG.edf...
EDF file detected
Setting channel info structure...
Creating raw.info structure...
Finally, we can plot the results:
df = pd.DataFrame(results)
fig, ax = plt.subplots(figsize=(6, 4))
colors = {True: 'tab:orange', False: 'tab:blue'}
markers = {n: m for n, m in zip(all_n_jobs, ['o', 'x', '.'])}
for (save, n_jobs), sub_df in df.groupby(['save', 'n_jobs']):
ax.scatter(x=sub_df['time'], y=sub_df['max_mem'], color=colors[save],
marker=markers[n_jobs], label=f'save={save}, n_jobs={n_jobs}')
ax.legend()
ax.set_xlabel('Execution time (s)')
ax.set_ylabel('Memory usage (MiB)')
ax.set_title(f'Loading and preprocessing {all_n_recs} recordings from Sleep '
'Physionet')
plt.show()
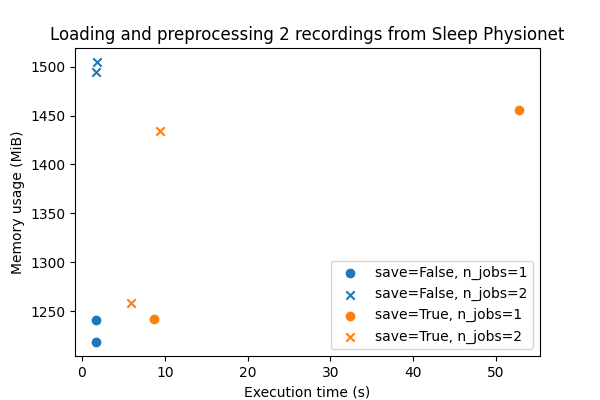
We see that parallel preprocessing without serialization (blue crosses) is faster than simple sequential processing (blue circles), however it uses more memory.
Combining parallel preprocessing and serialization (orange crosses) reduces memory usage significantly, however it increases run time by a few seconds. Depending on available resources (e.g. in limited memory settings), it might therefore be more advantageous to use both parallelization and serialization together.
Total running time of the script: (1 minutes 24.901 seconds)
Estimated memory usage: 95 MB